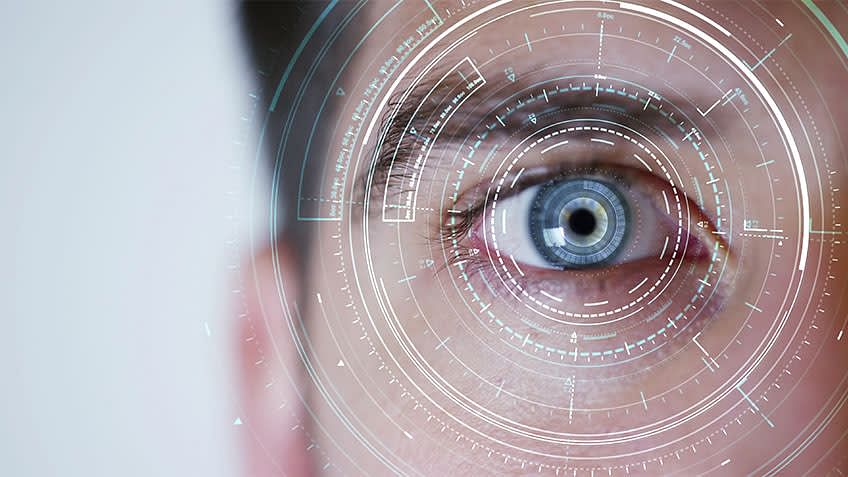
Image Processing Web App
- 1 minImage Processing
In today’s digital era, the power of visual data is undeniable. From enhancing photos to detecting objects, image processing has revolutionized the way we interpret and manipulate images. Image processing involves a series of operations that convert an image into a more useful form. It encompasses various tasks such as filtering, enhancing, and analyzing images to extract meaningful information.